안녕하세요.
‘수상한 김토끼’ 입니다.
이번 장에서는 Coherence를 IMDG로 사용할때 개발 및 테스트 단계에서 활용해 볼 수 있는 Eclipse 기반의 IMDG 테스트를 진행 해 보았습니다.
Coherence는 서버에 설치형으로 사용하는 제품이지만 단순 테스트나 기능확인등을 위해서 coherence.jar 파일만 추가하여 사용할 수있는 방법이 있어 그 내용을 기록 해 보았습니다.
이번장 진행에 참고한 Coherence Github 문서입니다.
https://github.com/oracle/coherenceGitHub – oracle/coherence: Oracle Coherence Community EditionOracle Coherence Community Edition. Contribute to oracle/coherence development by creating an account on GitHub.github.com
프로그래밍 방식의 Hello Coherence 예제
Eclipse에 Java 프로젝트를 생성한 후 저장소가 활성화된 Coherence 서버를 구성하여 간단한 Coherence 애플리케이션을 실행할 수 있습니다.
Coherence를 적용하기 위해서 필요한 단계는 Coherence.jar 파일을 클래스패스에 추가해 주는 과정 뿐이며
이를 손쉽게 사용하기 위해 Maven 프로젝트로 진행하였습니다.
실행환경은 Github문서에 명시 된 대로 Java 17버전에 Maven 3.9 버전을 사용하였습니다.
Hello Coherence 프로젝트 생성
1. 이클립스에서 maven-archetype-quickstart와 같은 아키타입을 사용하거나 일반 maven 프로젝트를 만듭니다.
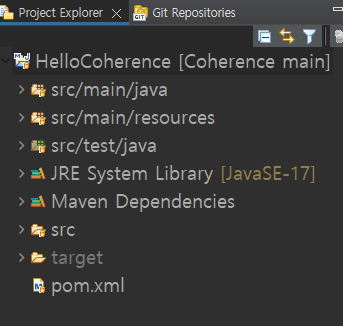
2. Coherence.jar 파일을 추가하기 위해 pom.xml 다음 항목을 추가하여 jar 파일을 다운로드 받습니다.
<dependency> <groupId>com.oracle.coherence.ce</groupId> <artifactId>coherence</artifactId> <version>22.06.10</version> </dependency>
추가 된 pom.xml 파일입니다.
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.oracle.coherence</groupId> <artifactId>HelloCoherence</artifactId> <version>0.0.1-SNAPSHOT</version> <name>HelloCoherence</name> <!-- FIXME change it to the project's website --> <url>http://www.example.com</url> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <maven.compiler.release>17</maven.compiler.release> </properties> <dependencyManagement> <dependencies> <dependency> <groupId>org.junit</groupId> <artifactId>junit-bom</artifactId> <version>5.11.0</version> <type>pom</type> <scope>import</scope> </dependency> </dependencies> </dependencyManagement> <dependencies> <dependency> <groupId>org.junit.jupiter</groupId> <artifactId>junit-jupiter-api</artifactId> <scope>test</scope> </dependency> <!-- Optionally: parameterized tests support --> <dependency> <groupId>org.junit.jupiter</groupId> <artifactId>junit-jupiter-params</artifactId> <scope>test</scope> </dependency> <!-- Oracle Coherence --> <dependency> <groupId>com.oracle.coherence.ce</groupId> <artifactId>coherence</artifactId> <version>22.06.10</version> </dependency> </dependencies> <build> <pluginManagement><!-- lock down plugins versions to avoid using Maven defaults (may be moved to parent pom) --> <plugins> <!-- clean lifecycle, see https://maven.apache.org/ref/current/maven-core/lifecycles.html#clean_Lifecycle --> <plugin> <artifactId>maven-clean-plugin</artifactId> <version>3.4.0</version> </plugin> <!-- default lifecycle, jar packaging: see https://maven.apache.org/ref/current/maven-core/default-bindings.html#Plugin_bindings_for_jar_packaging --> <plugin> <artifactId>maven-resources-plugin</artifactId> <version>3.3.1</version> </plugin> <plugin> <artifactId>maven-compiler-plugin</artifactId> <version>3.13.0</version> </plugin> <plugin> <artifactId>maven-surefire-plugin</artifactId> <version>3.3.0</version> </plugin> <plugin> <artifactId>maven-jar-plugin</artifactId> <version>3.4.2</version> </plugin> <plugin> <artifactId>maven-install-plugin</artifactId> <version>3.1.2</version> </plugin> <plugin> <artifactId>maven-deploy-plugin</artifactId> <version>3.1.2</version> </plugin> <!-- site lifecycle, see https://maven.apache.org/ref/current/maven-core/lifecycles.html#site_Lifecycle --> <plugin> <artifactId>maven-site-plugin</artifactId> <version>3.12.1</version> </plugin> <plugin> <artifactId>maven-project-info-reports-plugin</artifactId> <version>3.6.1</version> </plugin> </plugins> </pluginManagement> </build> </project>
이번 장에서는 IMDG 기능만 사용함으로 예제와 같이 Coherence CE 버전의 라이브러리를 사용했습니다만 추가 기능을 활용할 필요가 있는 경우 정식버전의 라이브러리를 사용하셔도 동일하게 적용 가능합니다.
3. src/main/java 경로에 main 클래스 파일을 작성 해 줍니다.
저는 HelloCoherence.java 파일을 다음과 같이 생성하였습니다.
package com.oracle.coherence.HelloCoherence; import com.tangosol.net.CacheFactory; import com.tangosol.net.NamedMap; public class HelloCoherence { public static void main(String[] args) { { NamedMap<String, String> map_1 = CacheFactory.getCache("welcomes"); NamedMap<String, String> map_2 = CacheFactory.getCache("goodbye"); System.out.printf("Accessing map \"%s\" containing %d entries\n", map_1.getName(), map_1.size()); System.out.printf("Accessing map \"%s\" containing %d entries\n", map_2.getName(), map_2.size()); map_1.put("english", "Hello"); map_1.put("spanish", "Hola"); map_1.put("french" , "Bonjour"); map_2.put("english", "ByeBye"); map_2.put("spanish", "Adios"); map_2.put("japan" , "Sayonara"); // list System.out.println("MapName:"+map_1.getName()); map_1.entrySet().forEach(System.out::println); // list2 System.out.println("MapName:"+map_2.getName()); map_2.entrySet().forEach(System.out::println); } } }
welcomes, goodbye라는 2개의 캐시를 생성하고 각각 3개의 데이터를 put 하고 꺼내어 출력하는 간단한 내용입니다.
이렇게 pom.xml 파일과 HelloCoherence.java 2개 파일을 추가한 후 Maven 빌드(package) 후 확인이 가능합니다.
작업 완료 후 Project Explorer는 아래와 같습니다.
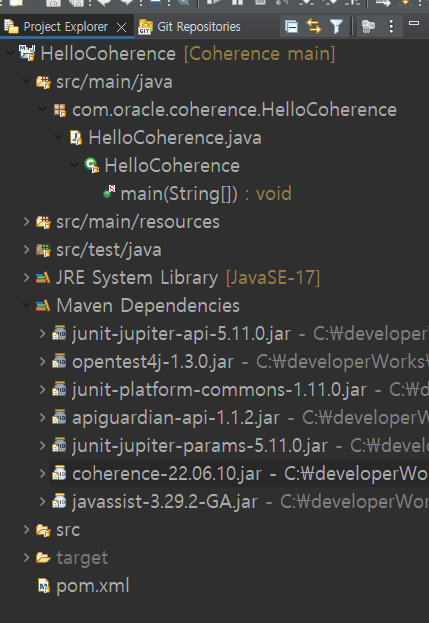
필요한 파일 작성이 마무리되었으니 Maven 빌드를 수행하여 프로젝트를 빌드 해 줍니다.
mvn package 명령으로 빌드를 실행합니다.
C:\developerWorks\eclipse-jee-2024-06-R-win32-x86_64\git\Coherence\HelloCoherence>mvn package [INFO] Scanning for projects... [INFO] [INFO] ----------------< com.oracle.coherence:HelloCoherence >----------------- [INFO] Building HelloCoherence 0.0.1-SNAPSHOT [INFO] from pom.xml [INFO] --------------------------------[ jar ]--------------------------------- [INFO] [INFO] --- resources:3.3.1:resources (default-resources) @ HelloCoherence --- [INFO] Copying 1 resource from src\main\resources to target\classes [INFO] [INFO] --- compiler:3.13.0:compile (default-compile) @ HelloCoherence --- [INFO] Nothing to compile - all classes are up to date. [INFO] [INFO] --- resources:3.3.1:testResources (default-testResources) @ HelloCoherence --- [INFO] skip non existing resourceDirectory C:\developerWorks\eclipse-jee-2024-06-R-win32-x86_64\git\Coherence\HelloCoherence\src\test\resources [INFO] [INFO] --- compiler:3.13.0:testCompile (default-testCompile) @ HelloCoherence --- [INFO] Nothing to compile - all classes are up to date. [INFO] [INFO] --- surefire:3.3.0:test (default-test) @ HelloCoherence --- [INFO] Using auto detected provider org.apache.maven.surefire.junitplatform.JUnitPlatformProvider [INFO] [INFO] ------------------------------------------------------- [INFO] T E S T S [INFO] ------------------------------------------------------- [INFO] Running com.oracle.coherence.HelloCoherence.AppTest [INFO] Tests run: 1, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.280 s -- in com.oracle.coherence.HelloCoherence.AppTest [INFO] [INFO] Results: [INFO] [INFO] Tests run: 1, Failures: 0, Errors: 0, Skipped: 0 [INFO] [INFO] [INFO] --- jar:3.4.2:jar (default-jar) @ HelloCoherence --- [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 8.646 s [INFO] Finished at: 2025-02-27T11:12:35+09:00 [INFO] ------------------------------------------------------------------------
우선 Coherence.jar 파일을 활용하여 캐시서버를 실행 후 main 클래스를 실행하여 동작여부를 확인할 수 있습니다.
터미널 혹은 cmd 창에서 프로젝트 디렉토리로 이동 후 다음 명령어로 캐시서버를 실행해 줍니다.
mvn exec:java -Dexec.mainClass="com.tangosol.net.DefaultCacheServer" &
동작이 완료되면 coherence.jar 파일을 활용해 캐시서버가 구동됩니다.
C:\developerWorks\eclipse-jee-2024-06-R-win32-x86_64\git\Coherence\HelloCoherence>mvn exec:java -Dexec.mainClass="com.tangosol.net.DefaultCacheServer" & [INFO] Scanning for projects... Downloading from central: https://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-metadata.xml Downloading from central: https://repo.maven.apache.org/maven2/org/codehaus/mojo/maven-metadata.xml Downloaded from central: https://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-metadata.xml (14 kB at 18 kB/s) Downloaded from central: https://repo.maven.apache.org/maven2/org/codehaus/mojo/maven-metadata.xml (21 kB at 25 kB/s) Downloading from central: https://repo.maven.apache.org/maven2/org/codehaus/mojo/exec-maven-plugin/maven-metadata.xml Downloaded from central: https://repo.maven.apache.org/maven2/org/codehaus/mojo/exec-maven-plugin/maven-metadata.xml (989 B at 27 kB/s) [INFO] [INFO] ----------------< com.oracle.coherence:HelloCoherence >----------------- [INFO] Building HelloCoherence 0.0.1-SNAPSHOT [INFO] from pom.xml [INFO] --------------------------------[ jar ]--------------------------------- [INFO] [INFO] --- exec:3.5.0:java (default-cli) @ HelloCoherence --- 2025-02-27 11:10:03.255/13.341 Oracle Coherence 22.06.10 <Info> (thread=com.tangosol.net.DefaultCacheServer.main(), member=n/a): Loaded operational configuration from "jar:file:/C:/Users/kami/.m2/repository/com/oracle/coherence/ce/coherence/22.06.10/coherence-22.06.10.jar!/tangosol-coherence.xml" 2025-02-27 11:10:03.657/13.665 Oracle Coherence 22.06.10 <Info> (thread=com.tangosol.net.DefaultCacheServer.main(), member=n/a): Loaded operational overrides from "jar:file:/C:/Users/kami/.m2/repository/com/oracle/coherence/ce/coherence/22.06.10/coherence-22.06.10.jar!/com/oracle/coherence/defaults/tangosol-coherence-override-dev.xml" 2025-02-27 11:10:03.718/13.727 Oracle Coherence 22.06.10 <Info> (thread=com.tangosol.net.DefaultCacheServer.main(), member=n/a): Loaded operational overrides from "file:/C:/developerWorks/eclipse-jee-2024-06-R-win32-x86_64/git/Coherence/HelloCoherence/target/classes/tangosol-coherence-override.xml" 2025-02-27 11:10:03.726/13.735 Oracle Coherence 22.06.10 <Info> (thread=com.tangosol.net.DefaultCacheServer.main(), member=n/a): Optional configuration override "cache-factory-config.xml" is not specified 2025-02-27 11:10:03.727/13.736 Oracle Coherence 22.06.10 <Info> (thread=com.tangosol.net.DefaultCacheServer.main(), member=n/a): Optional configuration override "cache-factory-builder-config.xml" is not specified 2025-02-27 11:10:03.727/13.737 Oracle Coherence 22.06.10 <Info> (thread=com.tangosol.net.DefaultCacheServer.main(), member=n/a): Optional configuration override "/custom-mbeans.xml" is not specified Oracle Coherence Version 22.06.10 Build 111636 Community Edition: Development mode Copyright (c) 2000, 2024, Oracle and/or its affiliates. All rights reserved. 2025-02-27 11:10:03.934/13.943 Oracle Coherence CE 22.06.10 <Info> (thread=com.tangosol.net.DefaultCacheServer.main(), member=n/a): Loaded cache configuration from "jar:file:/C:/Users/kami/.m2/repository/com/oracle/coherence/ce/coherence/22.06.10/coherence-22.06.10.jar!/com/oracle/coherence/defaults/coherence-cache-config.xml" 2025-02-27 11:10:05.411/15.420 Oracle Coherence CE 22.06.10 <D5> (thread=com.tangosol.net.DefaultCacheServer.main(), member=n/a): Created cache factory com.tangosol.net.ExtensibleConfigurableCacheFactory 2025-02-27 11:10:05.421/15.431 Oracle Coherence CE 22.06.10 <Info> (thread=com.tangosol.net.DefaultCacheServer.main(), member=n/a): ExtensibledConfigurableCacheFactory.activate() cache config with scope 2025-02-27 11:10:06.054/16.063 Oracle Coherence CE 22.06.10 <Info> (thread=com.tangosol.net.DefaultCacheServer.main(), member=n/a): Tracing support will not be enabled. The following dependencies appear to be missing: [opentracing-api, opentracing-util, opentracing-noop, opentracing-tracerresolver] 2025-02-27 11:10:06.154/16.163 Oracle Coherence CE 22.06.10 <Info> (thread=com.tangosol.net.DefaultCacheServer.main(), member=n/a): TCMP bound to /192.168.0.39:5159 using SystemDatagramSocketProvider 2025-02-27 11:10:09.714/19.722 Oracle Coherence CE 22.06.10 <Info> (thread=NameService:TcpAcceptor, member=n/a): TcpAcceptor now listening for connections on DESKTOP-1EOEL72:5159.3 2025-02-27 11:10:09.726/19.735 Oracle Coherence CE 22.06.10 <Info> (thread=Cluster, member=n/a): NameService now listening for connections on DESKTOP-1EOEL72:7574.3 2025-02-27 11:10:09.734/19.743 Oracle Coherence CE 22.06.10 <Info> (thread=Cluster, member=n/a): Created a new cluster "Kami-Coherence-Cluster" with Member(Id=1, Timestamp=2025-02-27 11:10:06.227, Address=192.168.0.39:5159, MachineId=46499, Location=process:12236, Role=CodehausPlexusClassworldsLauncherLauncher, Edition=Community Edition, Mode=Development, CpuCount=4, SocketCount=4) 2025-02-27 11:10:09.791/19.800 Oracle Coherence CE 22.06.10 <D4> (thread=Transport:TransportService, member=n/a): Initialized BufferManager to: NonDisposableBufferManager(delegate=DirectBufferManager(capacity=11MB, usage=0B..0B/0B, hit rate=0%, segment utilization=1KB(0%)/0, 2KB(0%)/0, 4KB(0%)/0, 8KB(0%)/0, 16KB(0%)/0, 32KB(0%)/0, 64KB(0%)/0, allocator=com.oracle.coherence.common.internal.io.SlabBufferManager$DirectBufferAllocator@7167844f)) 2025-02-27 11:10:09.801/19.809 Oracle Coherence CE 22.06.10 <D5> (thread=Transport:TransportService, member=n/a): Service TransportService is bound to tmb://192.168.0.39:5159.58228 2025-02-27 11:10:09.824/19.832 Oracle Coherence CE 22.06.10 <Info> (thread=Transport:TransportService, member=n/a): Service TransportService joined the cluster with senior service member 1 2025-02-27 11:10:09.838/19.847 Oracle Coherence CE 22.06.10 <Info> (thread=com.tangosol.net.DefaultCacheServer.main(), member=n/a): Started cluster Name=Kami-Coherence-Cluster, ClusterPort=7574 Group{Address=239.192.0.0, TTL=4} MasterMemberSet( ThisMember=Member(Id=1, Timestamp=2025-02-27 11:10:06.227, Address=192.168.0.39:5159, MachineId=46499, Location=process:12236, Role=CodehausPlexusClassworldsLauncherLauncher) OldestMember=Member(Id=1, Timestamp=2025-02-27 11:10:06.227, Address=192.168.0.39:5159, MachineId=46499, Location=process:12236, Role=CodehausPlexusClassworldsLauncherLauncher) ActualMemberSet=MemberSet(Size=1 Member(Id=1, Timestamp=2025-02-27 11:10:06.227, Address=192.168.0.39:5159, MachineId=46499, Location=process:12236, Role=CodehausPlexusClassworldsLauncherLauncher) ) MemberId|ServiceJoined|MemberState|Version|Edition 1|2025-02-27 11:10:06.227|JOINED|22.06.10|CE RecycleMillis=1200000 RecycleSet=MemberSet(Size=0 ) ) TcpRing{Connections=[]} IpMonitor{Addresses=0, Timeout=15s} 2025-02-27 11:10:09.856/19.866 Oracle Coherence CE 22.06.10 <Info> (thread=com.tangosol.net.DefaultCacheServer.main(), member=1): Loaded management configuration from "jar:file:/C:/Users/kami/.m2/repository/com/oracle/coherence/ce/coherence/22.06.10/coherence-22.06.10.jar!/com/oracle/coherence/defaults/management-config.xml" 2025-02-27 11:10:09.863/19.872 Oracle Coherence CE 22.06.10 <Info> (thread=Invocation:Management, member=1): Service Management joined the cluster with senior service member 1 2025-02-27 11:10:09.985/19.994 Oracle Coherence CE 22.06.10 <Info> (thread=com.tangosol.net.DefaultCacheServer.main(), member=1): Loaded Reporter configuration from "jar:file:/C:/Users/kami/.m2/repository/com/oracle/coherence/ce/coherence/22.06.10/coherence-22.06.10.jar!/reports/report-group.xml" 2025-02-27 11:10:10.088/20.097 Oracle Coherence CE 22.06.10 <Info> (thread=com.tangosol.net.DefaultCacheServer.main(), member=1): JMXConnectorServer now listening for connections on DESKTOP-1EOEL72:5160 2025-02-27 11:10:10.128/20.136 Oracle Coherence CE 22.06.10 <Info> (thread=com.tangosol.net.DefaultCacheServer.main(), member=1): Loaded Reporter configuration from "jar:file:/C:/Users/kami/.m2/repository/com/oracle/coherence/ce/coherence/22.06.10/coherence-22.06.10.jar!/reports/report-group.xml" 2025-02-27 11:10:10.754/20.763 Oracle Coherence CE 22.06.10 <Info> (thread=DistributedCache:PartitionedCache, member=1): Service PartitionedCache joined the cluster with senior service member 1 2025-02-27 11:10:10.771/20.781 Oracle Coherence CE 22.06.10 <D4> (thread=DistributedCache:PartitionedCache, member=1): Started DaemonPool "PartitionedCache": [DeamonCount=4, DaemonCountMax=2147483647, DaemonCountMin=4, Dynamic=true QueueSize=4, WorkSlots=4] 2025-02-27 11:10:10.806/20.815 Oracle Coherence CE 22.06.10 <Info> (thread=DistributedCache:PartitionedCache, member=1): Service PartitionedCache: sending PartitionConfig com.tangosol.coherence.component.util.daemon.queueProcessor.service.Grid.ConfigSync to all 2025-02-27 11:10:10.883/20.892 Oracle Coherence CE 22.06.10 <Info> (thread=DistributedCache:PartitionedCache, member=1): This member has become the distribution coordinator for MemberSet(Size=1 Member(Id=1, Timestamp=2025-02-27 11:10:06.227, Address=192.168.0.39:5159, MachineId=46499, Location=process:12236, Role=CodehausPlexusClassworldsLauncherLauncher) ) 2025-02-27 11:10:10.989/20.998 Oracle Coherence CE 22.06.10 <Info> (thread=DistributedCache:PartitionedCache, member=1): Partition ownership has stabilized with 1 nodes 2025-02-27 11:10:11.090/21.099 Oracle Coherence CE 22.06.10 <Info> (thread=PagedTopic:PartitionedTopic, member=1): Loaded POF configuration from "jar:file:/C:/Users/kami/.m2/repository/com/oracle/coherence/ce/coherence/22.06.10/coherence-22.06.10.jar!/com/oracle/coherence/defaults/pof-config.xml" 2025-02-27 11:10:11.104/21.112 Oracle Coherence CE 22.06.10 <Info> (thread=PagedTopic:PartitionedTopic, member=1): Loaded included POF configuration from "jar:file:/C:/Users/kami/.m2/repository/com/oracle/coherence/ce/coherence/22.06.10/coherence-22.06.10.jar!/coherence-pof-config.xml" 2025-02-27 11:10:11.557/21.565 Oracle Coherence CE 22.06.10 <Info> (thread=PagedTopic:PartitionedTopic, member=1): Service PartitionedTopic joined the cluster with senior service member 1 2025-02-27 11:10:11.746/21.754 Oracle Coherence CE 22.06.10 <D4> (thread=PagedTopic:PartitionedTopic, member=1): Started DaemonPool "PartitionedTopic": [DeamonCount=4, DaemonCountMax=2147483647, DaemonCountMin=4, Dynamic=true QueueSize=4, WorkSlots=4] 2025-02-27 11:10:11.749/21.757 Oracle Coherence CE 22.06.10 <Info> (thread=PagedTopic:PartitionedTopic, member=1): Service PartitionedTopic: sending PartitionConfig com.tangosol.coherence.component.util.daemon.queueProcessor.service.Grid.ConfigSync to all 2025-02-27 11:10:11.817/21.827 Oracle Coherence CE 22.06.10 <D4> (thread=PagedTopic:PartitionedTopic, member=1): Started DaemonPool "CoherenceCommonPool": [DeamonCount=4, DaemonCountMax=2147483647, DaemonCountMin=4, Dynamic=true QueueSize=4, WorkSlots=4] 2025-02-27 11:10:11.851/21.860 Oracle Coherence CE 22.06.10 <Info> (thread=PagedTopic:PartitionedTopic, member=1): This member has become the distribution coordinator for MemberSet(Size=1 Member(Id=1, Timestamp=2025-02-27 11:10:06.227, Address=192.168.0.39:5159, MachineId=46499, Location=process:12236, Role=CodehausPlexusClassworldsLauncherLauncher) ) 2025-02-27 11:10:11.889/21.898 Oracle Coherence CE 22.06.10 <Info> (thread=PagedTopic:PartitionedTopic, member=1): Partition ownership has stabilized with 1 nodes 2025-02-27 11:10:11.945/21.954 Oracle Coherence CE 22.06.10 <Info> (thread=Proxy:TcpAcceptor, member=1): TcpAcceptor now listening for connections on DESKTOP-1EOEL72:5159.38917 2025-02-27 11:10:11.948/21.957 Oracle Coherence CE 22.06.10 <D4> (thread=Proxy:TcpAcceptor, member=1): Started DaemonPool "Proxy:TcpAcceptor": [DeamonCount=1, DaemonCountMax=2147483647, DaemonCountMin=1, Dynamic=true QueueSize=1, WorkSlots=4] 2025-02-27 11:10:11.954/21.964 Oracle Coherence CE 22.06.10 <Info> (thread=Proxy, member=1): Service Proxy joined the cluster with senior service member 1 2025-02-27 11:10:11.966/21.975 Oracle Coherence CE 22.06.10 <Info> (thread=com.tangosol.net.DefaultCacheServer.main(), member=1): Started DefaultCacheServer Services ( ClusterService{Name=Cluster, State=(SERVICE_STARTED, STATE_JOINED), Id=0, OldestMemberId=1} TransportService{Name=TransportService, State=(SERVICE_STARTED), Id=1, OldestMemberId=1} InvocationService{Name=Management, State=(SERVICE_STARTED), Id=2, OldestMemberId=1, Serializer=java} PartitionedCache{Name=PartitionedCache, State=(SERVICE_STARTED), Id=3, OldestMemberId=1, LocalStorage=enabled, PartitionCount=257, BackupCount=1, AssignedPartitions=257, BackupPartitions=0, CoordinatorId=1, PersistenceMode=on-demand, Serializer=java} PagedTopic{Name=PartitionedTopic, State=(SERVICE_STARTED), Id=4, OldestMemberId=1, LocalStorage=enabled, PartitionCount=257, BackupCount=1, AssignedPartitions=257, BackupPartitions=0, CoordinatorId=1, PersistenceMode=on-demand, Serializer=safe-pof} ProxyService{Name=Proxy, State=(SERVICE_STARTED), Id=5, OldestMemberId=1, Serializer=java} )
캐시 서버가 구동되었으니 main 클래스를 수행하여 예제가 정상적으로 동작하는지 확인합니다.
터미널 혹은 cmd 창에서 프로젝트 디렉토리로 이동 후 다음 명령어로 main 클래스 실행해 줍니다.
mvn exec:java -Dexec.mainClass="com.oracle.coherence.HelloCoherence.HelloCoherence"
본인의 패키지+클래스명으로 mainClass 부분을 작성해 주시면 됩니다.
실행결과 아래와 같이 캐시 서버에 멤버로 접속하여 main 클래스의 내용이 수행된 것을 확인할 수 있습니다.
C:\developerWorks\eclipse-jee-2024-06-R-win32-x86_64\git\Coherence\HelloCoherence>mvn exec:java -Dexec.mainClass="com.oracle.coherence.HelloCoherence.HelloCoherence" [INFO] Scanning for projects... [INFO] [INFO] ----------------< com.oracle.coherence:HelloCoherence >----------------- [INFO] Building HelloCoherence 0.0.1-SNAPSHOT [INFO] from pom.xml [INFO] --------------------------------[ jar ]--------------------------------- [INFO] [INFO] --- exec:3.5.0:java (default-cli) @ HelloCoherence --- 2025-02-27 11:16:57.480/4.966 Oracle Coherence 22.06.10 <Info> (thread=com.oracle.coherence.HelloCoherence.HelloCoherence.main(), member=n/a): Loaded operational configuration from "jar:file:/C:/Users/kami/.m2/repository/com/oracle/coherence/ce/coherence/22.06.10/coherence-22.06.10.jar!/tangosol-coherence.xml" 2025-02-27 11:16:57.753/5.217 Oracle Coherence 22.06.10 <Info> (thread=com.oracle.coherence.HelloCoherence.HelloCoherence.main(), member=n/a): Loaded operational overrides from "jar:file:/C:/Users/kami/.m2/repository/com/oracle/coherence/ce/coherence/22.06.10/coherence-22.06.10.jar!/com/oracle/coherence/defaults/tangosol-coherence-override-dev.xml" 2025-02-27 11:16:57.799/5.263 Oracle Coherence 22.06.10 <Info> (thread=com.oracle.coherence.HelloCoherence.HelloCoherence.main(), member=n/a): Loaded operational overrides from "file:/C:/developerWorks/eclipse-jee-2024-06-R-win32-x86_64/git/Coherence/HelloCoherence/target/classes/tangosol-coherence-override.xml" 2025-02-27 11:16:57.805/5.269 Oracle Coherence 22.06.10 <Info> (thread=com.oracle.coherence.HelloCoherence.HelloCoherence.main(), member=n/a): Optional configuration override "cache-factory-config.xml" is not specified 2025-02-27 11:16:57.806/5.270 Oracle Coherence 22.06.10 <Info> (thread=com.oracle.coherence.HelloCoherence.HelloCoherence.main(), member=n/a): Optional configuration override "cache-factory-builder-config.xml" is not specified 2025-02-27 11:16:57.807/5.271 Oracle Coherence 22.06.10 <Info> (thread=com.oracle.coherence.HelloCoherence.HelloCoherence.main(), member=n/a): Optional configuration override "/custom-mbeans.xml" is not specified Oracle Coherence Version 22.06.10 Build 111636 Community Edition: Development mode Copyright (c) 2000, 2024, Oracle and/or its affiliates. All rights reserved. 2025-02-27 11:16:58.056/5.520 Oracle Coherence CE 22.06.10 <Info> (thread=com.oracle.coherence.HelloCoherence.HelloCoherence.main(), member=n/a): Loaded cache configuration from "jar:file:/C:/Users/kami/.m2/repository/com/oracle/coherence/ce/coherence/22.06.10/coherence-22.06.10.jar!/com/oracle/coherence/defaults/coherence-cache-config.xml" 2025-02-27 11:16:59.383/6.847 Oracle Coherence CE 22.06.10 <D5> (thread=com.oracle.coherence.HelloCoherence.HelloCoherence.main(), member=n/a): Created cache factory com.tangosol.net.ExtensibleConfigurableCacheFactory 2025-02-27 11:16:59.856/7.320 Oracle Coherence CE 22.06.10 <Info> (thread=com.oracle.coherence.HelloCoherence.HelloCoherence.main(), member=n/a): Tracing support will not be enabled. The following dependencies appear to be missing: [opentracing-api, opentracing-util, opentracing-noop, opentracing-tracerresolver] 2025-02-27 11:17:00.045/7.509 Oracle Coherence CE 22.06.10 <Info> (thread=com.oracle.coherence.HelloCoherence.HelloCoherence.main(), member=n/a): TCMP bound to /192.168.0.39:5234 using SystemDatagramSocketProvider 2025-02-27 11:17:00.412/7.875 Oracle Coherence CE 22.06.10 <Info> (thread=Cluster, member=n/a): Member(Id=1, Timestamp=2025-02-27 11:10:06.227, Address=192.168.0.39:5159, MachineId=46499, Location=process:12236, Role=CodehausPlexusClassworldsLauncherLauncher) joined Cluster with senior member 1 2025-02-27 11:17:00.421/7.885 Oracle Coherence CE 22.06.10 <Info> (thread=Cluster, member=n/a): This Member(Id=2, Timestamp=2025-02-27 11:17:00.205, Address=192.168.0.39:5234, MachineId=46499, Location=process:16492, Role=CodehausPlexusClassworldsLauncherLauncher, Edition=Community Edition, Mode=Development, CpuCount=4, SocketCount=4) joined cluster "Kami-Coherence-Cluster" with senior Member(Id=1, Timestamp=2025-02-27 11:10:06.227, Address=192.168.0.39:5159, MachineId=46499, Location=process:12236, Role=CodehausPlexusClassworldsLauncherLauncher, Edition=Community Edition, Mode=Development, CpuCount=4, SocketCount=4) 2025-02-27 11:17:00.697/8.162 Oracle Coherence CE 22.06.10 <D4> (thread=Transport:TransportService, member=n/a): Initialized BufferManager to: NonDisposableBufferManager(delegate=DirectBufferManager(capacity=13.6MB, usage=0B..0B/0B, hit rate=0%, segment utilization=1KB(0%)/0, 2KB(0%)/0, 4KB(0%)/0, 8KB(0%)/0, 16KB(0%)/0, 32KB(0%)/0, 64KB(0%)/0, allocator=com.oracle.coherence.common.internal.io.SlabBufferManager$DirectBufferAllocator@2718d348)) 2025-02-27 11:17:00.706/8.170 Oracle Coherence CE 22.06.10 <D5> (thread=Transport:TransportService, member=n/a): Service TransportService is bound to tmb://192.168.0.39:5234.57807 2025-02-27 11:17:00.771/8.235 Oracle Coherence CE 22.06.10 <Info> (thread=Transport:TransportService, member=n/a): Service TransportService joined the cluster with senior service member 1 2025-02-27 11:17:00.865/8.329 Oracle Coherence CE 22.06.10 <Info> (thread=SelectionService(channels=7, selector=MultiplexedSelector(sun.nio.ch.WEPollSelectorImpl@4ac22748), id=255983547), member=n/a): Connection established with tmb://192.168.0.39:5159.58228 2025-02-27 11:17:00.887/8.351 Oracle Coherence CE 22.06.10 <Info> (thread=com.oracle.coherence.HelloCoherence.HelloCoherence.main(), member=n/a): Started cluster Name=Kami-Coherence-Cluster, ClusterPort=7574 Group{Address=239.192.0.0, TTL=4} MasterMemberSet( ThisMember=Member(Id=2, Timestamp=2025-02-27 11:17:00.205, Address=192.168.0.39:5234, MachineId=46499, Location=process:16492, Role=CodehausPlexusClassworldsLauncherLauncher) OldestMember=Member(Id=1, Timestamp=2025-02-27 11:10:06.227, Address=192.168.0.39:5159, MachineId=46499, Location=process:12236, Role=CodehausPlexusClassworldsLauncherLauncher) ActualMemberSet=MemberSet(Size=2 Member(Id=1, Timestamp=2025-02-27 11:10:06.227, Address=192.168.0.39:5159, MachineId=46499, Location=process:12236, Role=CodehausPlexusClassworldsLauncherLauncher) Member(Id=2, Timestamp=2025-02-27 11:17:00.205, Address=192.168.0.39:5234, MachineId=46499, Location=process:16492, Role=CodehausPlexusClassworldsLauncherLauncher) ) MemberId|ServiceJoined|MemberState|Version|Edition 1|2025-02-27 11:10:06.227|JOINED|22.06.10|CE, 2|2025-02-27 11:17:00.205|JOINED|22.06.10|CE RecycleMillis=1200000 RecycleSet=MemberSet(Size=0 ) ) TcpRing{Connections=[1]} IpMonitor{Addresses=0, Timeout=15s} 2025-02-27 11:17:00.904/8.368 Oracle Coherence CE 22.06.10 <Info> (thread=com.oracle.coherence.HelloCoherence.HelloCoherence.main(), member=2): Loaded management configuration from "jar:file:/C:/Users/kami/.m2/repository/com/oracle/coherence/ce/coherence/22.06.10/coherence-22.06.10.jar!/com/oracle/coherence/defaults/management-config.xml" 2025-02-27 11:17:00.913/8.376 Oracle Coherence CE 22.06.10 <Info> (thread=Invocation:Management, member=2): Service Management joined the cluster with senior service member 1 2025-02-27 11:17:00.963/8.427 Oracle Coherence CE 22.06.10 <Info> (thread=com.oracle.coherence.HelloCoherence.HelloCoherence.main(), member=2): Loaded Reporter configuration from "jar:file:/C:/Users/kami/.m2/repository/com/oracle/coherence/ce/coherence/22.06.10/coherence-22.06.10.jar!/reports/report-group.xml" 2025-02-27 11:17:03.159/10.623 Oracle Coherence CE 22.06.10 <Info> (thread=DistributedCache:PartitionedCache, member=2): Service PartitionedCache joined the cluster with senior service member 1 Accessing map "welcomes" containing 0 entries Accessing map "goodbye" containing 0 entries MapName:welcomes ConverterEntry{Key="french", Value="Bonjour"} ConverterEntry{Key="spanish", Value="Hola"} ConverterEntry{Key="english", Value="Hello"} MapName:goodbye ConverterEntry{Key="japan", Value="Sayonara"} ConverterEntry{Key="spanish", Value="Adios"} ConverterEntry{Key="english", Value="ByeBye"} Interrupted PacketReceiver, Thread[PacketReceiver,10,Cluster] Interrupted PacketPublisher, Thread[PacketPublisher,10,Cluster] Interrupted TransportService, Thread[Transport:TransportService,10,Cluster] Interrupted InvocationService, Thread[Invocation:Management,10,Cluster] Interrupted EventDispatcher, Thread[Invocation:Management:EventDispatcher,10,Cluster] Interrupted PartitionedCache, Thread[DistributedCache:PartitionedCache,10,Cluster] Interrupted EventDispatcher, Thread[DistributedCache:PartitionedCache:EventDispatcher,10,Cluster] Interrupted IpMonitor, Thread[IpMonitor,6,Cluster] Interrupted Logger, Thread[Logger@9267279 22.06.10,3,com.oracle.coherence.HelloCoherence.HelloCoherence]
로그 중간의 출력부분에 아래 내용이 포함되었는지 확인을 통해 정상동작 여부를 알 수 있습니다.
MapName:welcomes ConverterEntry{Key="french", Value="Bonjour"} ConverterEntry{Key="spanish", Value="Hola"} ConverterEntry{Key="english", Value="Hello"} MapName:goodbye ConverterEntry{Key="japan", Value="Sayonara"} ConverterEntry{Key="spanish", Value="Adios"} ConverterEntry{Key="english", Value="ByeBye"}
이와같이 Coherence의 IMDG 기능은 Coherence.jar 파일만 클래스 패스에 추가해 주는 것만으로도 손쉽게 구현이 가능합니다. 다소 생소한 내용으로 활용 방법에 의문을 가지는 경우가 많은 것 같아 관련 내용을 포스팅 하게 되었습니다.
실제 업무에 적용하기 위해서는 여러 설정이 필요하며 이러한 설정은 ‘tangosol-coherence-override.xml’ 파일을 작성하여 Coherence.jar 파일 내부에 포함되어 있는 tangosol-coherence.xml 파일을 오버라이드 하여 적용이 가능합니다.
업무에 사용하기 위해서 우선적으로 캐시서버명, 타임아웃설정등이 정의 되어야 하고 본 예제와 달리 실제 서버에 설치 된 Coherence 캐시서버에 접속을 위한 WKA설정등이 포함되어야 하기 때문이며 관련내용은 tangosol-coherence-override 설정이라는 별도의 포스팅으로 작성해 보도록 하겠습니다.
이번 포스팅 내용은 간단히 jar 파일 추가 만으로 Coherence 캐시서버 구현 및 기능사용이 가능함을 알아보는 목적으로 이해하시면 좋을 것 같습니다.